How to Use Selenium for API and UI Test Automation Together?
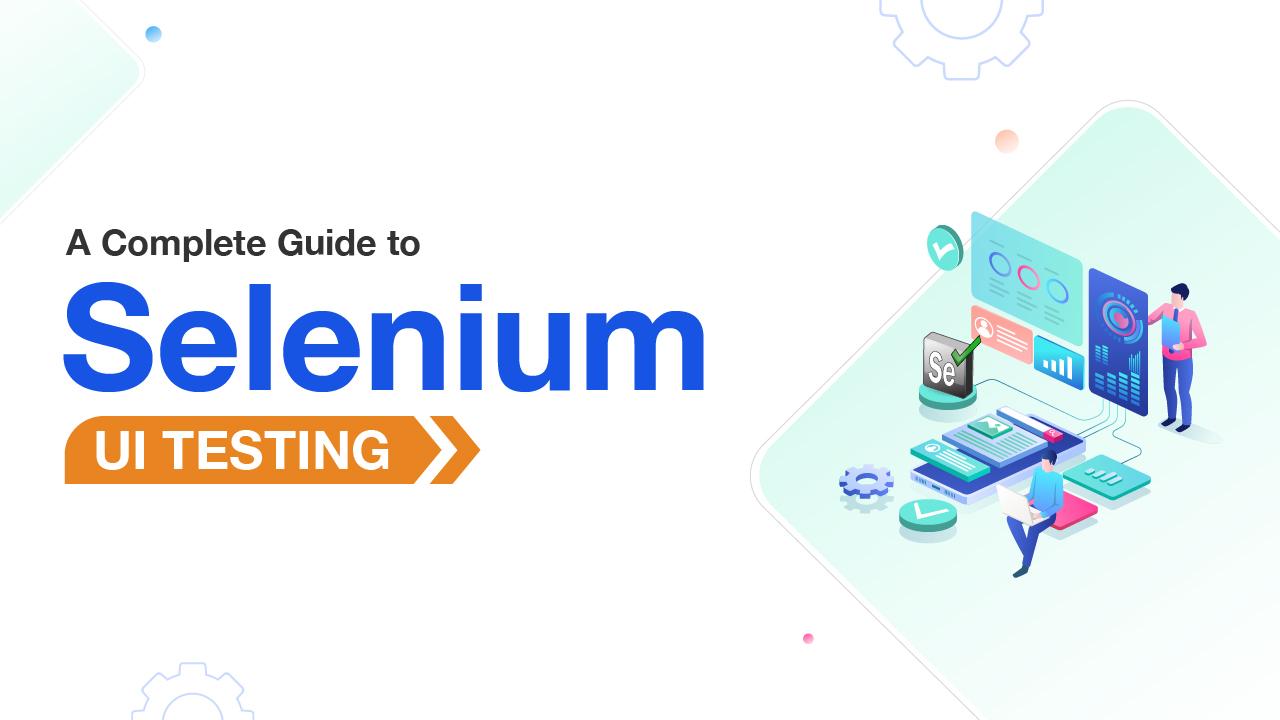
In the fast-paced world of software development, ensuring the quality of applications through automated testing has become a non-negotiable part of the development process. As developers and QA professionals work to deliver products that are efficient, reliable, and bug-free, two primary types of testing often come to the forefront: UI and API testing.
While both play crucial roles, most testing frameworks focus on either API testing or UI testing independently. But what if you could combine both using the powerful Selenium testing framework? By enrolling in a Selenium certification course, you can learn how to leverage Selenium for both UI and API testing, enhancing your skills and knowledge. In this blog post, we'll explore how to integrate Selenium for both API and UI test automation, making your testing process more efficient and comprehensive.
Selenium Automation Testing
Selenium is one of the most popular open-source frameworks for automating web browsers. Initially created for automating web applications for testing purposes, Selenium has expanded its capabilities and is now used for both UI and API testing.
Selenium is widely known for its ability to interact with browsers as a user would. It's designed to automate repetitive tasks in web applications, ensuring they function as expected. Whether you're running manual tests or developing automated ones, Selenium supports various programming languages like Java, Python, C#, and JavaScript, which makes it a flexible tool for automation testing.
By integrating API and UI testing into one framework, testers can leverage Selenium's power to test all aspects of a web application seamlessly.
What Is API Testing, and Why Is It Important?
API (Application Programming Interface) testing focuses on testing the endpoints of an application, ensuring that the interactions between various software systems work as expected. APIs are responsible for the communication between different software components, and testing them ensures that requests and responses are processed correctly.
Importance of API Testing:
-
Faster Feedback: API testing can be performed faster than UI tests since it doesn't require rendering a user interface.
-
Improved Reliability: Verifying that APIs return correct responses reduces the risk of errors when the front end (UI) and back end (API) interact.
-
Automation: Since APIs are typically designed to handle a large volume of requests, API testing can be automated to ensure every new version of the API works as expected.
What Is UI Testing, and Why Is It Important?
UI testing ensures that the application's user interface is functioning correctly. This testing verifies whether elements like buttons, links, forms, navigation, and other components work as intended from a user perspective.
Importance of UI Testing:
-
End-User Experience: Since UI is what users interact with, ensuring a smooth, bug-free user interface is critical for user satisfaction.
-
Visual Consistency: UI testing checks that all visual elements, like colors, fonts, and layouts, appear as expected across different browsers and devices.
-
Cross-Browser Testing: With Selenium's cross-browser support, you can ensure that your application performs consistently across multiple browsers.
Why Combine UI and API Testing Using Selenium?
Combining API and UI testing using Selenium provides several benefits:
-
End-to-End Coverage: By automating both API and UI tests, you cover the full stack of your application, from server-side logic to the user interface.
-
Efficiency: Running API tests first can help identify issues early in the back-end logic, and you can automate UI tests later to verify that the front-end components work as expected.
-
Reduced Redundancy: Instead of testing the UI and API separately, combining them can reduce repetitive test scripts and improve test maintenance.
-
Improved Accuracy: API and UI tests often work hand-in-hand. For example, if an API fails, it could directly affect the UI. By running them together, you can better diagnose issues that stem from the back-end and affect the front-end.
Setting Up Your Selenium Automation Testing Environment
Before you dive into writing tests, it’s essential to set up your Selenium environment. If you're new to Selenium, taking a Selenium course can help you understand the setup process and best practices. Here's a step-by-step guide to get started:
-
Install Java (or preferred programming language): Selenium supports Java, Python, C#, and other languages. Choose the one you're comfortable with.
-
Install Selenium WebDriver: The WebDriver is the core component of Selenium. You can download it from the official Selenium website and configure it for your programming language.
-
Choose Your IDE: A good IDE like Eclipse (for Java) or PyCharm (for Python) will help you manage your Selenium tests efficiently.
-
Set Up WebDriver for Browsers: Selenium supports multiple browsers like Chrome, Firefox, and Safari. Install the appropriate WebDriver binaries and configure them.
Once your environment is set up, you can begin automating both API and UI tests with Selenium.
Using Selenium for API Testing
While Selenium is primarily known for UI automation, it can also be used for API testing. To make this work, you'll need to integrate Selenium with tools that can handle HTTP requests, such as RestAssured (for Java) or Requests (for Python).
Here’s a simple example of how you might use RestAssured with Selenium for API testing:
Example: API Test with RestAssured (Java)
java
import io.restassured.RestAssured;
import io.restassured.response.Response;
import static io.restassured.RestAssured.*;
public class APITest {
public static void main(String[] args) {
// Set the base URL for the API
RestAssured.baseURI = "https://api.example.com";
// Send a GET request to the API endpoint
Response response = given()
.header("Authorization", "Bearer <token>")
.when()
.get("/endpoint")
.then()
.statusCode(200)
.extract()
.response();
// Print the response body
System.out.println(response.asString());
}
}
In this example, the API test sends a GET request to the specified endpoint, checks that the status code is 200 (OK), and extracts the response.
Using Selenium for UI Testing
Selenium makes UI testing straightforward by automating user interactions with web pages. You can automate tasks like clicking buttons, filling out forms, and verifying that elements are present or visible.
Here’s a simple example of a UI test using Selenium WebDriver in Java:
Example: UI Test with Selenium (Java)
java
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class UITest {
public static void main(String[] args) {
// Set the path to your WebDriver
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
// Initialize WebDriver
WebDriver driver = new ChromeDriver();
// Open the website
driver.get("https://www.example.com");
// Locate the login button and click it
driver.findElement(By.id("loginButton")).click();
// Fill out the login form
driver.findElement(By.id("username")).sendKeys("testuser");
driver.findElement(By.id("password")).sendKeys("password123");
// Submit the form
driver.findElement(By.id("loginSubmit")).click();
// Verify that login was successful
String pageTitle = driver.getTitle();
if (pageTitle.equals("Welcome Page")) {
System.out.println("Login successful!");
} else {
System.out.println("Login failed.");
}
// Close the browser
driver.quit();
}
}
This script opens a webpage, simulates a user login, and verifies if the login was successful by checking the page title.
How to Integrate Both API and UI Testing in Selenium
To combine both UI and API testing, you can follow this strategy: By enrolling in a Selenium certification, you’ll gain the skills needed to effectively integrate both types of testing. Here's how to get started:
-
Run API tests first: Test the core functionality through API tests to ensure that the server-side logic is correct.
-
Execute UI tests next: After verifying the back-end, run UI tests to confirm that the front-end elements behave as expected.
-
Report the results: Generate unified reports that highlight both API and UI test results, making it easier to trace any issues that occur.
You can structure your tests to follow a sequence like this:
-
Call the API to check if the application returns the expected data.
-
Use the data from the API to test the UI components.
-
Verify if the UI renders the data correctly and if any interaction works as expected.
Real-World Example: Automating UI and API Tests Together
Let’s consider a scenario where you’re testing a social media platform:
-
API Test: You start by testing the login API to ensure that users can authenticate and receive a token.
-
UI Test: After receiving the token, you use it in UI tests to log in through the website and ensure the user is redirected to the dashboard.
Both tests work in tandem, and you know that if either one fails, the issue likely lies either in the back-end API or the front-end UI.
Best Practices for Selenium Automation Testing
-
Modularize Test Scripts: Keep API and UI test scripts separate for better maintainability.
-
Use Waits: Always incorporate waits to handle dynamic elements.
-
Parallel Testing: Run tests in parallel across multiple browsers for efficiency.
-
Data-Driven Testing: Use data from APIs to drive UI tests, ensuring the tests are realistic.
-
Error Handling: Implement robust error handling to capture test failures and logs for easier debugging.
Key Takeaways
-
Selenium can be used for both API and UI automation, providing end-to-end test coverage.
-
API testing is crucial for verifying server-side functionality, while UI testing ensures a smooth user experience.
-
By combining both, you can identify issues that affect the entire application stack.
-
Selenium allows automation in multiple languages, giving you flexibility in your testing approach.
Conclusion
Automating both API and UI testing with Selenium is a powerful approach to ensure that your web applications function correctly from both the back-end and the front-end. By combining these testing strategies, you can improve efficiency, reduce redundancy, and enhance the accuracy of your testing process.
Are you ready to take your Selenium testing skills to the next level? Consider enrolling in a Selenium certification course or Selenium training to learn how to effectively automate both API and UI testing and boost your career in automation testing.